useCalendar
Provides the behavior and accessibility implementation for a calendar component. A calendar displays one or more date grids and allows users to select a single date.
install | yarn add react-aria |
---|---|
version | 3.38.0 |
usage | import {useCalendar} from 'react-aria' |
API#
useCalendar<T extends >(
(props: <T>,
, state:
)):
useCalendarGrid(
(props: ,
, state:
| |
)):
useCalendarCell(
props: ,
state:
| | ,
ref: <HTMLElement
| | null>
):
Features#
There is no standalone calendar element in HTML. <input type="date">
is close, but this is very limited in functionality, lacking in internationalization capabilities, inconsistent between browsers, and difficult to style. useCalendar
helps achieve accessible and international calendar components that can be styled as needed.
- Flexible – Display one or more months at once, or a custom time range for use cases like a week view. Minimum and maximum values, unavailable dates, and non-contiguous selections are supported as well.
- International – Support for 13 calendar systems used around the world, including Gregorian, Buddhist, Islamic, Persian, and more. Locale-specific formatting, number systems, and right-to-left support are available as well.
- Accessible – Calendar cells can be navigated and selected using the keyboard, and localized screen reader messages are included to announce when the selection and visible date range change.
- Customizable – As with all of React Aria, the DOM structure and styling of all elements can be fully customized.
Read our blog post for more details about the internationalization, accessibility, and user experience features implemented by useCalendar
.
Anatomy#
A calendar consists of a grouping element containing one or more date grids (e.g. months), and a previous and next button for navigating between date ranges. Each calendar grid consists of cells containing button elements that can be pressed and navigated to using the arrow keys to select a date.
useCalendar#
useCalendar
returns props that you should spread onto the appropriate elements:
Name | Type | Description |
calendarProps | DOMAttributes | Props for the calendar grouping element. |
nextButtonProps |
| Props for the next button. |
prevButtonProps |
| Props for the previous button. |
errorMessageProps | DOMAttributes | Props for the error message element, if any. |
title | string | A description of the visible date range, for use in the calendar title. |
useCalendarGrid#
useCalendarGrid
returns props for an individual grid of dates, such as one month, along with a list of formatted weekday names in the current locale for use during rendering:
Name | Type | Description |
gridProps | DOMAttributes | Props for the date grid element (e.g. <table> ). |
headerProps | DOMAttributes | Props for the grid header element (e.g. <thead> ). |
weekDays | string[] | A list of week day abbreviations formatted for the current locale, typically used in column headers. |
useCalendarCell#
useCalendarCell
returns props for an individual cell, along with states and information useful during rendering:
Name | Type | Description |
cellProps | DOMAttributes | Props for the grid cell element (e.g. <td> ). |
buttonProps | DOMAttributes | Props for the button element within the cell. |
isPressed | boolean | Whether the cell is currently being pressed. |
isSelected | boolean | Whether the cell is selected. |
isFocused | boolean | Whether the cell is focused. |
isDisabled | boolean | Whether the cell is disabled, according to the calendar's |
isUnavailable | boolean | Whether the cell is unavailable, according to the calendar's Note that because they are focusable, unavailable dates must meet a 4.5:1 color contrast ratio, as defined by WCAG. |
isOutsideVisibleRange | boolean | Whether the cell is outside the visible range of the calendar. For example, dates before the first day of a month in the same week. |
isInvalid | boolean | Whether the cell is part of an invalid selection. |
formattedDate | string | The day number formatted according to the current locale. |
State is managed by the hook from
@react-stately/calendar
. The state object should be passed as an option to useCalendar
, useCalendarGrid
, and useCalendarCell
.
Note that much of this anatomy is shared with range calendars. The only difference is that useCalendarState
is used instead of useRangeCalendarState
, and useCalendar
is used instead of useRangeCalendar
.
Date and time values#
Dates are represented in many different ways by cultures around the world. This includes differences in calendar systems, date formatting, numbering systems, weekday and weekend rules, and much more. When building applications that support users around the world, it is important to handle these aspects correctly for each locale.
uses the @internationalized/date library to represent dates and times. This package provides a library of objects and functions to perform date and time related manipulation, queries, and conversions that work across locales and calendars. Date and time objects can be converted to and from native JavaScript
Date
objects or ISO 8601 strings. See the documentation, or the examples below for more details.
requires a
createCalendar
function to be provided, which is used to implement date manipulation across multiple calendar systems. The default implementation in @internationalized/date
includes all supported calendar systems. While this library is quite small (8 kB minified + Brotli), you can reduce its bundle size further by providing your own implementation that includes only your supported calendars. See below for an example.
Example#
A Calendar
consists of three components: the main calendar wrapper element with previous and next buttons for navigating, one or more CalendarGrid
components to display each month, and CalendarCell
components for each date cell. We'll go through them one by one.
For simplicity, this example only displays a single month at a time. See the styled examples section for more examples with multiple months, as well as other time ranges like weeks.
import {useCalendar, useLocale} from 'react-aria';
import {useCalendarState} from 'react-stately';
import {createCalendar} from '@internationalized/date';
// Reuse the Button from your component library. See below for details.
import {Button} from 'your-component-library';
function Calendar(props) {
let { locale } = useLocale();
let state = useCalendarState({
...props,
locale,
createCalendar
});
let { calendarProps, prevButtonProps, nextButtonProps, title } = useCalendar(
props,
state
);
return (
<div {...calendarProps} className="calendar">
<div className="header">
<h2>{title}</h2>
<Button {...prevButtonProps}><</Button>
<Button {...nextButtonProps}>></Button>
</div>
<CalendarGrid state={state} firstDayOfWeek={props.firstDayOfWeek} />
</div>
);
}
import {useCalendar, useLocale} from 'react-aria';
import {useCalendarState} from 'react-stately';
import {createCalendar} from '@internationalized/date';
// Reuse the Button from your component library. See below for details.
import {Button} from 'your-component-library';
function Calendar(props) {
let { locale } = useLocale();
let state = useCalendarState({
...props,
locale,
createCalendar
});
let {
calendarProps,
prevButtonProps,
nextButtonProps,
title
} = useCalendar(props, state);
return (
<div {...calendarProps} className="calendar">
<div className="header">
<h2>{title}</h2>
<Button {...prevButtonProps}><</Button>
<Button {...nextButtonProps}>></Button>
</div>
<CalendarGrid
state={state}
firstDayOfWeek={props.firstDayOfWeek}
/>
</div>
);
}
import {
useCalendar,
useLocale
} from 'react-aria';
import {useCalendarState} from 'react-stately';
import {createCalendar} from '@internationalized/date';
// Reuse the Button from your component library. See below for details.
import {Button} from 'your-component-library';
function Calendar(
props
) {
let { locale } =
useLocale();
let state =
useCalendarState({
...props,
locale,
createCalendar
});
let {
calendarProps,
prevButtonProps,
nextButtonProps,
title
} = useCalendar(
props,
state
);
return (
<div
{...calendarProps}
className="calendar"
>
<div className="header">
<h2>{title}</h2>
<Button
{...prevButtonProps}
>
<
</Button>
<Button
{...nextButtonProps}
>
>
</Button>
</div>
<CalendarGrid
state={state}
firstDayOfWeek={props
.firstDayOfWeek}
/>
</div>
);
}
CalendarGrid#
The CalendarGrid
component will be responsible for rendering an individual month. It is a separate component so that you can render more than one month at a time if you like. It's rendered as an HTML <table>
element, and React Aria takes care of adding the proper ARIA roles and event handlers to make it behave as an ARIA grid. You can use the arrow keys to navigate between cells, and the Enter key to select a date.
The state.getDatesInWeek
function returns the dates in each week of the month. Note that this always includes 7 values, but some of them may be null, which indicates that the date doesn't exist within the calendar system. You should render a placeholder <td>
element in this case so that the cells line up correctly.
Note: this component is the same as the CalendarGrid
component shown in the useRangeCalendar docs, and you can reuse it between both Calendar
and RangeCalendar
.
import {useCalendarGrid} from 'react-aria';
import {getWeeksInMonth} from '@internationalized/date';
function CalendarGrid({ state, ...props }) {
let { locale } = useLocale();
let { gridProps, headerProps, weekDays } = useCalendarGrid(props, state);
// Get the number of weeks in the month so we can render the proper number of rows.
let weeksInMonth = getWeeksInMonth(
state.visibleRange.start,
locale,
props.firstDayOfWeek
);
return (
<table {...gridProps}>
<thead {...headerProps}>
<tr>
{weekDays.map((day, index) => <th key={index}>{day}</th>)}
</tr>
</thead>
<tbody>
{[...new Array(weeksInMonth).keys()].map((weekIndex) => (
<tr key={weekIndex}>
{state.getDatesInWeek(weekIndex).map((date, i) => (
date
? (
<CalendarCell
key={i}
state={state}
date={date}
/>
)
: <td key={i} />
))}
</tr>
))}
</tbody>
</table>
);
}
import {useCalendarGrid} from 'react-aria';
import {getWeeksInMonth} from '@internationalized/date';
function CalendarGrid({ state, ...props }) {
let { locale } = useLocale();
let { gridProps, headerProps, weekDays } =
useCalendarGrid(props, state);
// Get the number of weeks in the month so we can render the proper number of rows.
let weeksInMonth = getWeeksInMonth(
state.visibleRange.start,
locale,
props.firstDayOfWeek
);
return (
<table {...gridProps}>
<thead {...headerProps}>
<tr>
{weekDays.map((day, index) => (
<th key={index}>{day}</th>
))}
</tr>
</thead>
<tbody>
{[...new Array(weeksInMonth).keys()].map(
(weekIndex) => (
<tr key={weekIndex}>
{state.getDatesInWeek(weekIndex).map((
date,
i
) => (
date
? (
<CalendarCell
key={i}
state={state}
date={date}
/>
)
: <td key={i} />
))}
</tr>
)
)}
</tbody>
</table>
);
}
import {useCalendarGrid} from 'react-aria';
import {getWeeksInMonth} from '@internationalized/date';
function CalendarGrid(
{ state, ...props }
) {
let { locale } =
useLocale();
let {
gridProps,
headerProps,
weekDays
} = useCalendarGrid(
props,
state
);
// Get the number of weeks in the month so we can render the proper number of rows.
let weeksInMonth =
getWeeksInMonth(
state.visibleRange
.start,
locale,
props
.firstDayOfWeek
);
return (
<table
{...gridProps}
>
<thead
{...headerProps}
>
<tr>
{weekDays.map((
day,
index
) => (
<th
key={index}
>
{day}
</th>
))}
</tr>
</thead>
<tbody>
{[...new Array(
weeksInMonth
).keys()].map(
(weekIndex) => (
<tr
key={weekIndex}
>
{state
.getDatesInWeek(
weekIndex
).map((
date,
i
) => (
date
? (
<CalendarCell
key={i}
state={state}
date={date}
/>
)
: (
<td
key={i}
/>
)
))}
</tr>
)
)}
</tbody>
</table>
);
}
CalendarCell#
Finally, the CalendarCell
component renders an individual cell in a calendar. It consists of two elements: a <td>
to represent the grid cell, and a <div>
to represent a button that can be clicked to select the date. The useCalendarCell
hook also returns the formatted date string in the current locale, as well as some information about the cell's state that can be useful for styling. See above for details.
Note: this component is the same as the CalendarCell
component shown in the useRangeCalendar docs, and you can reuse it between both Calendar
and RangeCalendar
.
import {useCalendarCell} from 'react-aria';
function CalendarCell({ state, date }) {
let ref = React.useRef(null);
let {
cellProps,
buttonProps,
isSelected,
isOutsideVisibleRange,
isDisabled,
isUnavailable,
formattedDate
} = useCalendarCell({ date }, state, ref);
return (
<td {...cellProps}>
<div
{...buttonProps}
ref={ref}
hidden={isOutsideVisibleRange}
className={`cell `}
>
{formattedDate}
</div>
</td>
);
}
import {useCalendarCell} from 'react-aria';
function CalendarCell({ state, date }) {
let ref = React.useRef(null);
let {
cellProps,
buttonProps,
isSelected,
isOutsideVisibleRange,
isDisabled,
isUnavailable,
formattedDate
} = useCalendarCell({ date }, state, ref);
return (
<td {...cellProps}>
<div
{...buttonProps}
ref={ref}
hidden={isOutsideVisibleRange}
className={`cell `}
>
{formattedDate}
</div>
</td>
);
}
import {useCalendarCell} from 'react-aria';
function CalendarCell(
{ state, date }
) {
let ref = React.useRef(
null
);
let {
cellProps,
buttonProps,
isSelected,
isOutsideVisibleRange,
isDisabled,
isUnavailable,
formattedDate
} = useCalendarCell(
{ date },
state,
ref
);
return (
<td {...cellProps}>
<div
{...buttonProps}
ref={ref}
hidden={isOutsideVisibleRange}
className={`cell `}
>
{formattedDate}
</div>
</td>
);
}
That's it! Now we can render an example of our Calendar
component in action.
<Calendar aria-label="Event date" />
<Calendar aria-label="Event date" />
<Calendar aria-label="Event date" />
Show CSS
.calendar {
width: 220px;
}
.header {
display: flex;
align-items: center;
gap: 4px;
margin: 0 8px;
}
.header h2 {
flex: 1;
margin: 0;
}
.calendar table {
width: 100%;
}
.cell {
cursor: default;
text-align: center;
}
.selected {
background: var(--blue);
color: white;
}
.unavailable {
color: var(--spectrum-global-color-red-600);
}
.disabled {
color: gray;
}
.calendar {
width: 220px;
}
.header {
display: flex;
align-items: center;
gap: 4px;
margin: 0 8px;
}
.header h2 {
flex: 1;
margin: 0;
}
.calendar table {
width: 100%;
}
.cell {
cursor: default;
text-align: center;
}
.selected {
background: var(--blue);
color: white;
}
.unavailable {
color: var(--spectrum-global-color-red-600);
}
.disabled {
color: gray;
}
.calendar {
width: 220px;
}
.header {
display: flex;
align-items: center;
gap: 4px;
margin: 0 8px;
}
.header h2 {
flex: 1;
margin: 0;
}
.calendar table {
width: 100%;
}
.cell {
cursor: default;
text-align: center;
}
.selected {
background: var(--blue);
color: white;
}
.unavailable {
color: var(--spectrum-global-color-red-600);
}
.disabled {
color: gray;
}
Button#
The Button
component is used in the above example to navigate between months. It is built using the useButton hook, and can be shared with many other components.
Show code
import {useButton} from 'react-aria';
function Button(props) {
let ref = React.useRef(null);
let { buttonProps } = useButton(props, ref);
return <button {...buttonProps} ref={ref}>{props.children}</button>;
}
import {useButton} from 'react-aria';
function Button(props) {
let ref = React.useRef(null);
let { buttonProps } = useButton(props, ref);
return (
<button {...buttonProps} ref={ref}>
{props.children}
</button>
);
}
import {useButton} from 'react-aria';
function Button(props) {
let ref = React.useRef(
null
);
let { buttonProps } =
useButton(
props,
ref
);
return (
<button
{...buttonProps}
ref={ref}
>
{props.children}
</button>
);
}
Styled Examples#
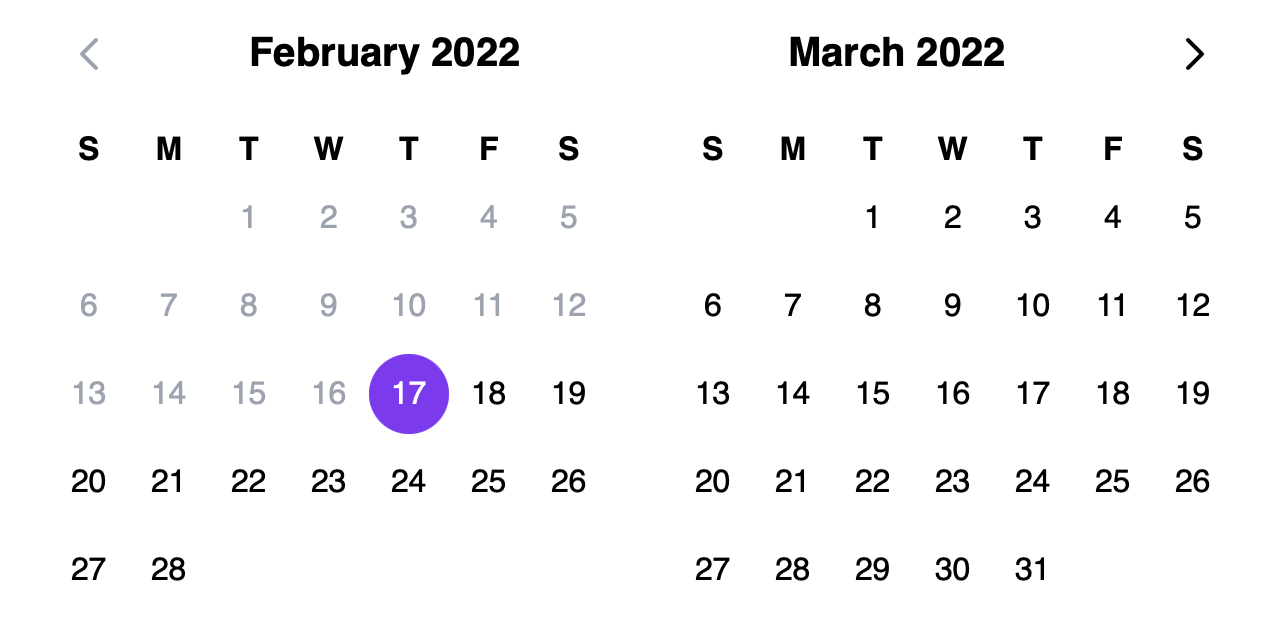
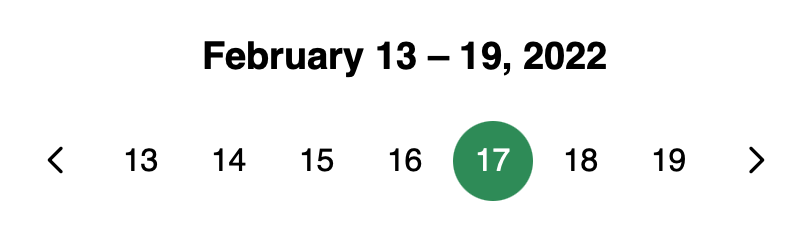
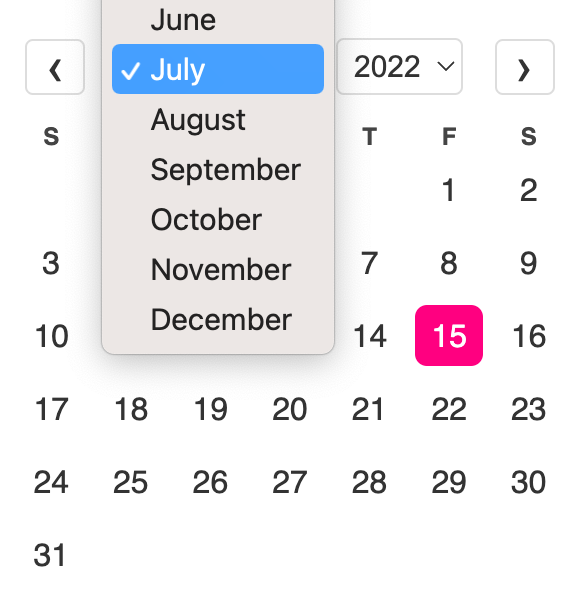
Usage#
The following examples show how to use the Calendar
component created in the above example.
Value#
A Calendar
has no selection by default. An initial, uncontrolled value can be provided to the Calendar
using the defaultValue
prop. Alternatively, a controlled value can be provided using the value
prop.
Date values are provided using objects in the @internationalized/date package. This library handles correct international date manipulation across calendars, time zones, and other localization concerns.
useCalendar
supports values with both date and time components, but only allows users to modify the date. By default, useCalendar
will emit objects in the
onChange
event, but if a or
object is passed as the
value
or defaultValue
, values of that type will be emitted, changing only the date and preserving the time components.
import {parseDate} from '@internationalized/date';
function Example() {
let [value, setValue] = React.useState(parseDate('2020-02-03'));
return (
<div style={{display: 'flex', gap: 20, flexWrap: 'wrap'}}>
<Calendar
aria-label="Date (uncontrolled)"
defaultValue={parseDate('2020-02-03')} />
<Calendar
aria-label="Date (controlled)"
value={value}
onChange={setValue} />
</div>
);
}
import {parseDate} from '@internationalized/date';
function Example() {
let [value, setValue] = React.useState(
parseDate('2020-02-03')
);
return (
<div
style={{ display: 'flex', gap: 20, flexWrap: 'wrap' }}
>
<Calendar
aria-label="Date (uncontrolled)"
defaultValue={parseDate('2020-02-03')}
/>
<Calendar
aria-label="Date (controlled)"
value={value}
onChange={setValue}
/>
</div>
);
}
import {parseDate} from '@internationalized/date';
function Example() {
let [value, setValue] =
React.useState(
parseDate(
'2020-02-03'
)
);
return (
<div
style={{
display: 'flex',
gap: 20,
flexWrap: 'wrap'
}}
>
<Calendar
aria-label="Date (uncontrolled)"
defaultValue={parseDate(
'2020-02-03'
)}
/>
<Calendar
aria-label="Date (controlled)"
value={value}
onChange={setValue}
/>
</div>
);
}
Events#
useCalendar
accepts an onChange
prop which is triggered whenever a date is selected by the user. The example below uses onChange
to update a separate element with a formatted version of the date in the user's locale. This is done by converting the date to a native JavaScript Date
object to pass to the formatter.
import {useDateFormatter} from 'react-aria';
import {getLocalTimeZone} from '@internationalized/date';
function Example() {
let [date, setDate] = React.useState(parseDate('2022-07-04'));
let formatter = useDateFormatter({ dateStyle: 'full' });
return (
<>
<Calendar aria-label="Event date" value={date} onChange={setDate} />
<p>Selected date: {formatter.format(date.toDate(getLocalTimeZone()))}</p>
</>
);
}
import {useDateFormatter} from 'react-aria';
import {getLocalTimeZone} from '@internationalized/date';
function Example() {
let [date, setDate] = React.useState(
parseDate('2022-07-04')
);
let formatter = useDateFormatter({ dateStyle: 'full' });
return (
<>
<Calendar
aria-label="Event date"
value={date}
onChange={setDate}
/>
<p>
Selected date:{' '}
{formatter.format(date.toDate(getLocalTimeZone()))}
</p>
</>
);
}
import {useDateFormatter} from 'react-aria';
import {getLocalTimeZone} from '@internationalized/date';
function Example() {
let [date, setDate] =
React.useState(
parseDate(
'2022-07-04'
)
);
let formatter =
useDateFormatter({
dateStyle: 'full'
});
return (
<>
<Calendar
aria-label="Event date"
value={date}
onChange={setDate}
/>
<p>
Selected date:
{' '}
{formatter
.format(
date.toDate(
getLocalTimeZone()
)
)}
</p>
</>
);
}
International calendars#
useCalendar
supports selecting dates in many calendar systems used around the world, including Gregorian, Hebrew, Indian, Islamic, Buddhist, and more. Dates are automatically displayed in the appropriate calendar system for the user's locale. The calendar system can be overridden using the Unicode calendar locale extension, passed to the I18nProvider component.
Selected dates passed to onChange
always use the same calendar system as the value
or defaultValue
prop. If no value
or defaultValue
is provided, then dates passed to onChange
are always in the Gregorian calendar since this is the most commonly used. This means that even though the user selects dates in their local calendar system, applications are able to deal with dates from all users consistently.
The below example displays a Calendar
in the Hindi language, using the Indian calendar. Dates emitted from onChange
are in the Gregorian calendar.
import {I18nProvider} from 'react-aria';
function Example() {
let [date, setDate] = React.useState(null);
return (
<I18nProvider locale="hi-IN-u-ca-indian">
<Calendar aria-label="Date" value={date} onChange={setDate} />
<p>Selected date: {date?.toString()}</p>
</I18nProvider>
);
}
import {I18nProvider} from 'react-aria';
function Example() {
let [date, setDate] = React.useState(null);
return (
<I18nProvider locale="hi-IN-u-ca-indian">
<Calendar
aria-label="Date"
value={date}
onChange={setDate}
/>
<p>Selected date: {date?.toString()}</p>
</I18nProvider>
);
}
import {I18nProvider} from 'react-aria';
function Example() {
let [date, setDate] =
React.useState(null);
return (
<I18nProvider locale="hi-IN-u-ca-indian">
<Calendar
aria-label="Date"
value={date}
onChange={setDate}
/>
<p>
Selected date:
{' '}
{date
?.toString()}
</p>
</I18nProvider>
);
}
Validation#
By default, useCalendar
allows selecting any date. The minValue
and maxValue
props can also be used to prevent the user from selecting dates outside a certain range.
This example only accepts dates after today.
import {today} from '@internationalized/date';
<Calendar
aria-label="Appointment date"
minValue={today(getLocalTimeZone())}
/>
import {today} from '@internationalized/date';
<Calendar
aria-label="Appointment date"
minValue={today(getLocalTimeZone())}
/>
import {today} from '@internationalized/date';
<Calendar
aria-label="Appointment date"
minValue={today(
getLocalTimeZone()
)}
/>
Unavailable dates#
useCalendar
supports marking certain dates as unavailable. These dates remain focusable with the keyboard so that navigation is consistent, but cannot be selected by the user. In this example, they are displayed in red. The isDateUnavailable
prop accepts a callback that is called to evaluate whether each visible date is unavailable.
This example includes multiple unavailable date ranges, e.g. dates when no appointments are available. In addition, all weekends are unavailable. The minValue
prop is also used to prevent selecting dates before today.
import {useLocale} from 'react-aria';
import {isWeekend, today} from '@internationalized/date';
function Example() {
let now = today(getLocalTimeZone());
let disabledRanges = [
[now, now.add({ days: 5 })],
[now.add({ days: 14 }), now.add({ days: 16 })],
[now.add({ days: 23 }), now.add({ days: 24 })]
];
let { locale } = useLocale();
let isDateUnavailable = (date) =>
isWeekend(date, locale) ||
disabledRanges.some((interval) =>
date.compare(interval[0]) >= 0 && date.compare(interval[1]) <= 0
);
return (
<Calendar
aria-label="Appointment date"
minValue={today(getLocalTimeZone())}
isDateUnavailable={isDateUnavailable}
/>
);
}
import {useLocale} from 'react-aria';
import {isWeekend, today} from '@internationalized/date';
function Example() {
let now = today(getLocalTimeZone());
let disabledRanges = [
[now, now.add({ days: 5 })],
[now.add({ days: 14 }), now.add({ days: 16 })],
[now.add({ days: 23 }), now.add({ days: 24 })]
];
let { locale } = useLocale();
let isDateUnavailable = (date) =>
isWeekend(date, locale) ||
disabledRanges.some((interval) =>
date.compare(interval[0]) >= 0 &&
date.compare(interval[1]) <= 0
);
return (
<Calendar
aria-label="Appointment date"
minValue={today(getLocalTimeZone())}
isDateUnavailable={isDateUnavailable}
/>
);
}
import {useLocale} from 'react-aria';
import {
isWeekend,
today
} from '@internationalized/date';
function Example() {
let now = today(
getLocalTimeZone()
);
let disabledRanges = [
[
now,
now.add({
days: 5
})
],
[
now.add({
days: 14
}),
now.add({
days: 16
})
],
[
now.add({
days: 23
}),
now.add({
days: 24
})
]
];
let { locale } =
useLocale();
let isDateUnavailable =
(date) =>
isWeekend(
date,
locale
) ||
disabledRanges
.some((
interval
) =>
date.compare(
interval[0]
) >= 0 &&
date.compare(
interval[1]
) <= 0
);
return (
<Calendar
aria-label="Appointment date"
minValue={today(
getLocalTimeZone()
)}
isDateUnavailable={isDateUnavailable}
/>
);
}
Controlling the focused date#
By default, the selected date is focused when a Calendar
first mounts. If no value
or defaultValue
prop is provided, then the current date is focused. However, useCalendar
supports controlling which date is focused using the focusedValue
and onFocusChange
props. This also determines which month is visible. The defaultFocusedValue
prop allows setting the initial focused date when the Calendar
first mounts, without controlling it.
This example focuses July 1, 2021 by default. The user may change the focused date, and the onFocusChange
event updates the state. Clicking the button resets the focused date back to the initial value.
import {CalendarDate} from '@internationalized/date';
function Example() {
let defaultDate = new CalendarDate(2021, 7, 1);
let [focusedDate, setFocusedDate] = React.useState(defaultDate);
return (
<div style={{ flexDirection: 'column', alignItems: 'start', gap: 20 }}>
<button onClick={() => setFocusedDate(defaultDate)}>
Reset focused date
</button>
<Calendar focusedValue={focusedDate} onFocusChange={setFocusedDate} />
</div>
);
}
import {CalendarDate} from '@internationalized/date';
function Example() {
let defaultDate = new CalendarDate(2021, 7, 1);
let [focusedDate, setFocusedDate] = React.useState(
defaultDate
);
return (
<div
style={{
flexDirection: 'column',
alignItems: 'start',
gap: 20
}}
>
<button onClick={() => setFocusedDate(defaultDate)}>
Reset focused date
</button>
<Calendar
focusedValue={focusedDate}
onFocusChange={setFocusedDate}
/>
</div>
);
}
import {CalendarDate} from '@internationalized/date';
function Example() {
let defaultDate =
new CalendarDate(
2021,
7,
1
);
let [
focusedDate,
setFocusedDate
] = React.useState(
defaultDate
);
return (
<div
style={{
flexDirection:
'column',
alignItems:
'start',
gap: 20
}}
>
<button
onClick={() =>
setFocusedDate(
defaultDate
)}
>
Reset focused
date
</button>
<Calendar
focusedValue={focusedDate}
onFocusChange={setFocusedDate}
/>
</div>
);
}
Disabled#
The isDisabled
boolean prop makes the Calendar disabled. Cells cannot be focused or selected.
<Calendar aria-label="Event date" isDisabled />
<Calendar aria-label="Event date" isDisabled />
<Calendar
aria-label="Event date"
isDisabled
/>
Read only#
The isReadOnly
boolean prop makes the Calendar's value immutable. Unlike isDisabled
, the Calendar remains focusable.
<Calendar
aria-label="Event date"
value={today(getLocalTimeZone())}
isReadOnly
/>
<Calendar
aria-label="Event date"
value={today(getLocalTimeZone())}
isReadOnly
/>
<Calendar
aria-label="Event date"
value={today(
getLocalTimeZone()
)}
isReadOnly
/>
Custom first day of week#
By default, the first day of the week is automatically set based on the current locale. This can be changed by setting the firstDayOfWeek
prop to 'sun'
, 'mon'
, 'tue'
, 'wed'
, 'thu'
, 'fri'
, or 'sat'
.
<Calendar
aria-label="Event date"
value={today(getLocalTimeZone())}
firstDayOfWeek="mon"
/>
<Calendar
aria-label="Event date"
value={today(getLocalTimeZone())}
firstDayOfWeek="mon"
/>
<Calendar
aria-label="Event date"
value={today(
getLocalTimeZone()
)}
firstDayOfWeek="mon"
/>
Labeling#
An aria-label must be provided to the Calendar
for accessibility. If it is labeled by a separate element, an aria-labelledby
prop must be provided using the id
of the labeling element instead.
Internationalization#
In order to internationalize a Calendar
, a localized string should be passed to the aria-label
prop. For languages that are read right-to-left (e.g. Hebrew and Arabic), keyboard navigation is automatically flipped. Ensure that your CSS accounts for this as well. Dates are automatically formatted using the current locale.
Advanced topics#
Reducing bundle size#
In the example above, the function from the @internationalized/date package is passed to the
hook. This function receives a calendar identifier string, and provides
instances to React Stately, which are used to implement date manipulation.
By default, this includes all calendar systems supported by @internationalized/date
. However, if your application supports a more limited set of regions, or you know you will only be picking dates in a certain calendar system, you can reduce your bundle size by providing your own implementation of createCalendar
that includes a subset of these Calendar
implementations.
For example, if your application only supports Gregorian dates, you could implement a createCalendar
function like this:
import {useLocale} from 'react-aria';
import {useCalendarState} from 'react-stately';
import {GregorianCalendar} from '@internationalized/date';
function createCalendar(identifier) {
switch (identifier) {
case 'gregory':
return new GregorianCalendar();
default:
throw new Error(`Unsupported calendar `);
}
}
function Calendar(props) {
let { locale } = useLocale();
let state = useCalendarState({
...props,
locale,
createCalendar
});
// ...
}
import {useLocale} from 'react-aria';
import {useCalendarState} from 'react-stately';
import {GregorianCalendar} from '@internationalized/date';
function createCalendar(identifier) {
switch (identifier) {
case 'gregory':
return new GregorianCalendar();
default:
throw new Error(`Unsupported calendar `);
}
}
function Calendar(props) {
let { locale } = useLocale();
let state = useCalendarState({
...props,
locale,
createCalendar
});
// ...
}
import {useLocale} from 'react-aria';
import {useCalendarState} from 'react-stately';
import {GregorianCalendar} from '@internationalized/date';
function createCalendar(
identifier
) {
switch (identifier) {
case 'gregory':
return new GregorianCalendar();
default:
throw new Error(
`Unsupported calendar
`);
}
}
function Calendar(
props
) {
let { locale } =
useLocale();
let state =
useCalendarState({
...props,
locale,
createCalendar
});
// ...
}
This way, only GregorianCalendar
is imported, and the other calendar implementations can be tree-shaken.
See the Calendar documentation in @internationalized/date
to learn more about the supported calendar systems, and a list of string identifiers.